Junit4 断言案例
1 概述
JUnit 为所有原始类型 和 对象 以及 (原始或对象的)数组提供了重载的断言方法 。参数顺序是期望值,然后是实际值。可选地,第一个参数可以是失败时输出的String消息。
有一个稍微不同的断言,assertThat具有可选失败消息的参数,实际值和Matcher对象。请注意,与其他assert方法相比,期望值和实际值是相反的。
让我们创建一个简单的maven项目并演示所有断言方法。
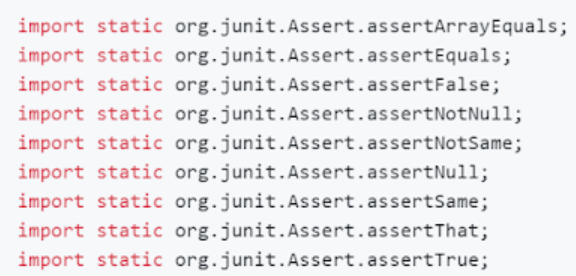
2 创建简单的Maven项目
让我们创建一个简单的Maven项目。通过执行以下命令创建简单的Maven项目:
mvn archetype:generate
-DgroupId=org.yourcompany.project
-DartifactId=application
在maven项目构建成功后,maven将创建默认文件夹结构。
2.1 项目包装结构
- src/main/java:Java源代码包和类
- src/main/resources:非Java资源,例如属性文件和Spring配置
Test
- src/test/java :测试源代码包和类
- src/test/resources :非Java资源,例如属性文件和Spring配置
── pom.xml
└── src
├── main
│ ├── java
│ │ └── com
│ │ └── yiidian
│ │ └── junit
│ ├── resources
└── test
├── java
│ └── com
│ └── yiidian
│ └── junit
│ └── AssertTests.java
└── resources
3 更新pom.xml文件中的JUnit依赖关系
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
4 创建一个AssertTests.java并运行测试
import static org.hamcrest.CoreMatchers.allOf;
import static org.hamcrest.CoreMatchers.anyOf;
import static org.hamcrest.CoreMatchers.both;
import static org.hamcrest.CoreMatchers.containsString;
import static org.hamcrest.CoreMatchers.equalTo;
import static org.hamcrest.CoreMatchers.everyItem;
import static org.hamcrest.CoreMatchers.hasItems;
import static org.hamcrest.CoreMatchers.not;
import static org.hamcrest.CoreMatchers.sameInstance;
import static org.hamcrest.CoreMatchers.startsWith;
import static org.junit.Assert.assertArrayEquals;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertFalse;
import static org.junit.Assert.assertNotNull;
import static org.junit.Assert.assertNotSame;
import static org.junit.Assert.assertNull;
import static org.junit.Assert.assertSame;
import static org.junit.Assert.assertThat;
import static org.junit.Assert.assertTrue;
import java.util.Arrays;
/**
* 一点教程网: http://www.yiidian.com
*/
import org.hamcrest.core.CombinableMatcher;
import org.junit.Test;
public class AssertTests {
@Test
public void testAssertArrayEquals() {
byte[] expected = "trial".getBytes();
byte[] actual = "trial".getBytes();
assertArrayEquals("failure - byte arrays not same", expected, actual);
}
@Test
public void testAssertEquals() {
assertEquals("failure - strings are not equal", "text", "text");
}
@Test
public void testAssertFalse() {
assertFalse("failure - should be false", false);
}
@Test
public void testAssertNotNull() {
assertNotNull("should not be null", new Object());
}
@Test
public void testAssertNotSame() {
assertNotSame("should not be same Object", new Object(), new Object());
}
@Test
public void testAssertNull() {
assertNull("should be null", null);
}
@Test
public void testAssertSame() {
Integer aNumber = Integer.valueOf(768);
assertSame("should be same", aNumber, aNumber);
}
// JUnit Matchers assertThat
@Test
public void testAssertThatBothContainsString() {
assertThat("albumen", both(containsString("a")).and(containsString("b")));
}
@Test
public void testAssertThatHasItems() {
assertThat(Arrays.asList("one", "two", "three"), hasItems("one", "three"));
}
@Test
public void testAssertThatEveryItemContainsString() {
assertThat(Arrays.asList(new String[] { "fun", "ban", "net" }), everyItem(containsString("n")));
}
// Core Hamcrest Matchers with assertThat
@Test
public void testAssertThatHamcrestCoreMatchers() {
assertThat("good", allOf(equalTo("good"), startsWith("good")));
assertThat("good", not(allOf(equalTo("bad"), equalTo("good"))));
assertThat("good", anyOf(equalTo("bad"), equalTo("good")));
assertThat(7, not(CombinableMatcher.<Integer> either(equalTo(3)).or(equalTo(4))));
assertThat(new Object(), not(sameInstance(new Object())));
}
@Test
public void testAssertTrue() {
assertTrue("failure - should be true", true);
}
}
4.1 运行测试
从命令行使用maven运行测试
mvn -Dtest=AssertTests test
4.2 输出
[INFO] Scanning for projects...
[INFO]
[INFO] ------------------------------------------------------------------------
[INFO] Building junit-assertions-examples 0.0.1-SNAPSHOT
[INFO] ------------------------------------------------------------------------
[INFO]
[INFO] --- maven-resources-plugin:2.6:resources (default-resources) @ junit-assertions-examples ---
[WARNING] Using platform encoding (Cp1252 actually) to copy filtered resources, i.e. build is platform dependent!
[INFO] Copying 0 resource
[INFO]
[INFO] --- maven-compiler-plugin:3.1:compile (default-compile) @ junit-assertions-examples ---
[INFO] Nothing to compile - all classes are up to date
[INFO]
[INFO] --- maven-resources-plugin:2.6:testResources (default-testResources) @ junit-assertions-examples ---
[WARNING] Using platform encoding (Cp1252 actually) to copy filtered resources, i.e. build is platform dependent!
[INFO] Copying 0 resource
[INFO]
[INFO] --- maven-compiler-plugin:3.1:testCompile (default-testCompile) @ junit-assertions-examples ---
[INFO] Nothing to compile - all classes are up to date
[INFO]
[INFO] --- maven-surefire-plugin:2.12.4:test (default-test) @ junit-assertions-examples ---
[INFO] Surefire report directory: E:\Git_Work\junit-developers-guide\junit-assertions-examples\target\surefire-reports
-------------------------------------------------------
T E S T S
-------------------------------------------------------
Running com.developersguide.junit.assetions.AssertTests
Tests run: 12, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.084 sec
Results :
Tests run: 12, Failures: 0, Errors: 0, Skipped: 0
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 1.641 s
[INFO] Finished at: 2018-06-27T22:12:19+05:30
[INFO] Final Memory: 6M/19M
[INFO] ------------------------------------------------------------------------
5 结论
在本指南中,我们通过创建一个简单的maven项目了解了JUnit 4 Framework提供的所有断言。您可以创建更多的测试方法,并应用这些断言以获得实践经验。
热门文章
优秀文章